Barcodes in MS VB.NET and C#
A word of caution: You might be tempted to import the ActiveX Control BarCodeWiz.dll by clicking on Tools > Choose Toolbox Items... > COM Components > Browse... .
However, Visual Studio does not import the properties correctly. When accessing the Picture property in a project compiled for x64 or Any CPU, an exception "A generic error occurred in GDI+" is thrown.
Instead, use the supplied Interop Assemblies as outlined in the steps below to ensure compatibility with both 32 and 64-bit projects.
Step 1. Add AxBarCodeWiz to Toolbox
- Click on Tools > Choose Toolbox Items...
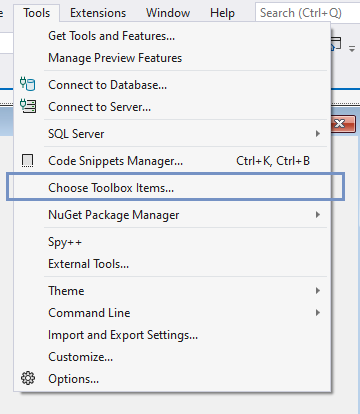
- With .NET Framework Components selected, click Browse...
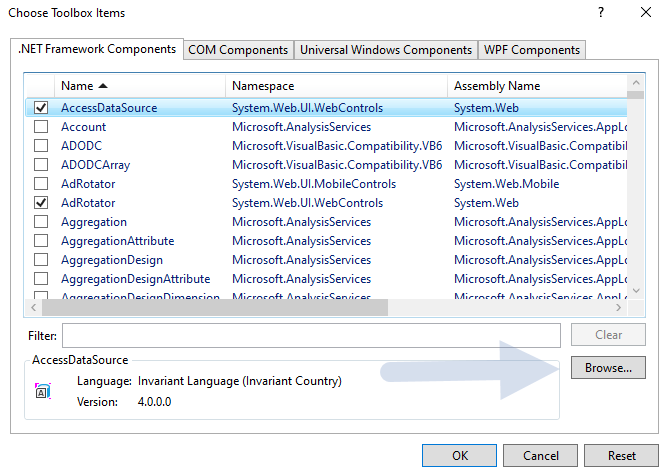
- Select AxBARCODEWIZLib.dll. The default location of the file is:
C:\Program Files\BarCodeWiz\BarCodeWiz ActiveX Control\Dll\Interop\4.0\AxBARCODEWIZLib.dll
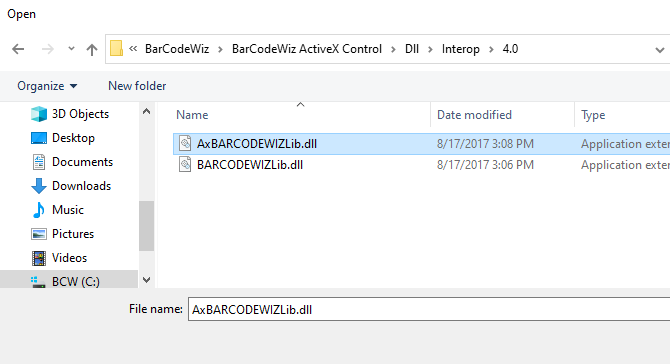
Step 2. Add reference to BARCODEWIZLib.dll
- Click Project > Add Project Reference...
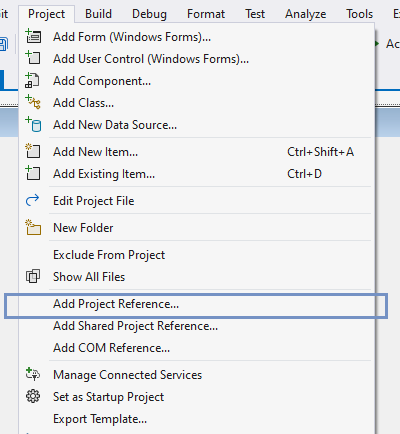
- Click on the Browse tab and select BARCODEWIZLib.dll. The default location of the file is:
C:\Program Files\BarCodeWiz\BarCodeWiz ActiveX Control\Dll\Interop\4.0\BARCODEWIZLib.dll
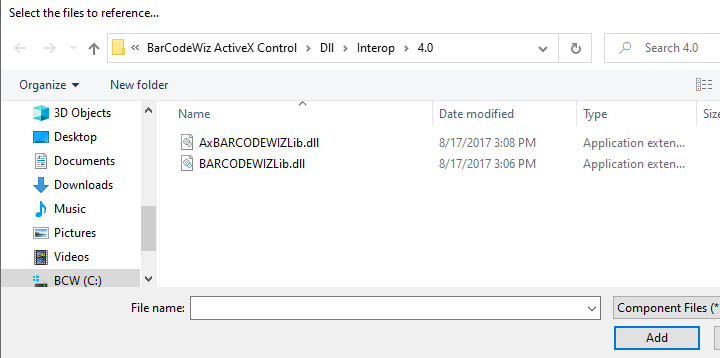
Step 3. Edit Properties
- In Solution Explorer click on BARCODEWIZLib and set Embed Interop Types to False and Copy Local to True.
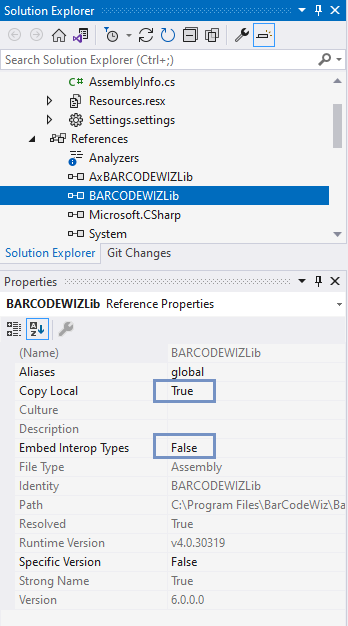
Ready
- AxBarCodeWiz is now available in the toolbox. Drag it onto a form as you would any other control.
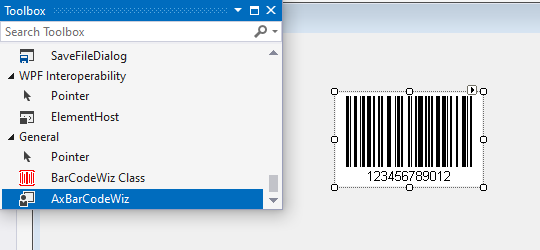
VB.NET Example:
Public Class Form1
Private Sub Button1_Click(ByVal sender As System.Object, _
ByVal e As System.EventArgs) Handles Button1.Click
'Set the barcode properties.
AxBarCodeWiz1.Symbology = BARCODEWIZLib.enumSYMBOLOGY.Code_128_Auto
AxBarCodeWiz1.Barcode = "HELLO 123"
Dim pd As New System.Drawing.Printing.PrintDocument
AddHandler pd.PrintPage, AddressOf pd_PrintPage
Dim pp As New PrintPreviewDialog
pp.Document = pd
pp.ShowDialog(Me)
End Sub
Private Sub pd_PrintPage(ByVal sender As Object, _
ByVal ev As System.Drawing.Printing.PrintPageEventArgs)
'Add the barcode at position (100,100).
ev.Graphics.DrawImage(AxBarCodeWiz1.Picture, 100, 100)
'No more pages, let's print.
ev.HasMorePages = False
End Sub
End Class
C# Example
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Text;
using System.Windows.Forms;
using System.Drawing.Printing;
namespace WindowsApplication1
{
public partial class Form1 : Form
{
public Form1()
{
InitializeComponent();
}
private void button1_Click(object sender, EventArgs e)
{
// Set the barcode properties.
axBarCodeWiz1.Symbology = BARCODEWIZLib.enumSYMBOLOGY.Code_128_Auto;
axBarCodeWiz1.Barcode = "HELLO 123";
PrintPreviewDialog pp = new PrintPreviewDialog();
PrintDocument pd = new PrintDocument();
pd.PrintPage += new PrintPageEventHandler(pd_PrintPage);
pp.Document = pd;
pp.ShowDialog(this);
}
private void pd_PrintPage(object sender, PrintPageEventArgs ev)
{
// Add the barcode at position (100,100).
ev.Graphics.DrawImage(axBarCodeWiz1.Picture, 100, 100);
// No more pages, let's print.
ev.HasMorePages = false;
}
}
}
See More
- See more complete examples under:
Documents\BarCodeWiz Examples\Barcode ActiveX Control\